It’s one of the “Design and Implementation” category patterns. This pattern was first described by Sam Newman. The definition of this pattern is given below.
Create separate backend services to be consumed by specific frontend applications or interfaces. This pattern is useful when you want to avoid customizing a single backend for multiple interfaces.
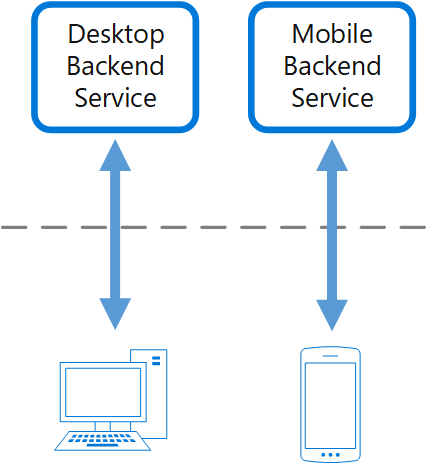
Consider that you have an application and it’s initially targeted for Desktop web UI users, so you develop a backend service that provides the features needed for that UI. After few months or years, your application’s user grows and now you want to develop a Mobile application which is going to access the same back end service.
Desktop Application UI and mobile application UI both have different capabilities so you might have different display limitations and due to those, you might want to display more data on the Desktop Web UI and less data on the Mobile application UI. So you are going to update the existing backend service to support both Desktop UI and mobile application UI behaviors. This will make the backend service be a bottleneck in the development process because it has to support various types of requirements. So to avoid this kind of problem, we can create a separate backend service for Desktop UI and separate one for Mobile UI.
Code duplication across services is highly likely when using this pattern. So think about keeping the common logic and business logic in a separate layer.
Advantages of this pattern:
Each backend is specific to one interface. So the code base becomes smaller and easily maintainable.
We can use different languages or frameworks to develop the back end services.
If there are lots of users accessing mobile UI, then we can selectively scale the Mobile backend service alone.
When not to use this pattern:
If your application has both Desktop UI and mobile application UI and both of those use the same backend service and no custom backend code specific to display, then you should avoid using this pattern.
When only one interface is used to interact with the backend.